`s
* Ensure contrast, focusability, and proper labels
* Use `alt` text and avoid relying on color alone
* Test your sections with real assistive tools
Design for everyone. Accessible sections create better experiences — for every shopper.
---
---
url: /building-theme/adding-layouts.md
---
# Adding Layouts
In Bagisto Visual, layouts define the **main HTML structure** of your pages.
Layouts are Blade files that act as **wrappers** for all templates and sections.
They typically include shared elements like the header, footer, meta tags, and asset loading.
## Creating the `default` Layout
The most important layout file is **`default.blade.php`**.
Create the file inside:
```text
resources/views/layouts/default.blade.php
```
Example content for a basic `default.blade.php`:
```blade
Awesome Theme
@bagistoVite(['resources/assets/css/app.css', 'resources/assets/js/app.js'])
@stack('meta')
@stack('styles')
@visual_layout_content
@stack('scripts')
```
* `
` loads the header component.
* `
` loads the footer component.
* `@visual_layout_content` renders the page content (templates and sections).
* `@bagisto_vite([...], 'awesome-theme')` includes theme assets correctly.
## Header and Footer Components
Create reusable header and footer components:
```text
resources/views/components/header.blade.php
resources/views/components/footer.blade.php
```
Basic `header.blade.php` example:
```blade
```
Basic `footer.blade.php` example:
```blade
```
✅ Both components are automatically registered under the `shop::` namespace.
## Views Namespace
All views inside your theme are automatically registered under two namespaces:
* `shop::` (default and recommended)
* `awesome-theme::` (theme code namespace)
For example:
* `resources/views/layouts/default.blade.php` → `shop::layouts.default`
* `resources/views/components/header.blade.php` → `
`
* `resources/views/pages/home.blade.php` → `shop::pages.home`
Components must be included using **Blade component syntax**:
```blade
```
You could also use the `awesome-theme::` namespace (example: `
`),
but to keep it **simple and standard**, we always use the **`shop::` namespace** in this documentation.
## Checking Your Layout
After setting up your layout and components:
1. Make sure you have created:
* `resources/views/layouts/default.blade.php`
* `resources/views/components/header.blade.php`
* `resources/views/components/footer.blade.php`
2. Go to your store **homepage**.
You should now see the default layout rendered —
showing your basic **header**, **main area**, and **footer**.
✅
If you see this, it means your layout setup is working correctly!
# Next Steps
Now that your layout is ready, you can move on to:
* [Creating Templates](./adding-templates.md)
* [Creating Sections](./adding-sections/overview.md)
---
---
url: /building-theme/adding-templates.md
---
# Adding Templates
Templates define the structure of store pages in Bagisto Visual.
They control how sections are arranged and rendered inside the main layout.
Templates can be created using two formats:
* **Blade templates** (`.blade.php`) — simple static content or basic Blade directives
* **JSON templates** (`.json` or `.yaml`) — fully dynamic, section-driven layouts for the Visual Editor
At this stage, we will start with a Blade template for simplicity.
## Creating a Blade Template
Blade templates are simple files that contain the page content.
They are injected into the layout through `@visual_layout_content`.
To create a homepage template:
1. Create a new file:
```text
resources/views/templates/index.blade.php
```
2. Add the following example content:
```blade
Welcome to Awesome Theme
Your new store is ready to be customized.
```
This content will be rendered inside your theme’s default layout.
There is no need to use `@extends` or `@section`.
## Viewing the Template
Once the template file is created:
* Visit your store homepage in the browser.
* You should see the header and footer rendered from the layout.
* The page body will display the content from `index.blade.php`.
At this stage, no sections are included yet.
Templates are static until sections are added.
## JSON Templates
Bagisto Visual also supports creating templates using JSON files.
JSON templates enable merchants to visually edit pages, add sections dynamically, and control page structure without touching code.
The structure and behavior of JSON templates are explained in the [JSON Template](../core-concepts/templates/json-template.md) documentation.
In the next chapter, we will cover:
* How to create sections
* How to use sections inside JSON templates to build dynamic pages
For now, starting with a simple Blade template is sufficient to initialize your theme.
## Summary
* Templates define page content and are rendered inside layouts.
* Blade templates are static and easy to start with.
* JSON templates enable full dynamic editing and will be introduced after learning about sections.
* Templates must be placed in `resources/views/templates/`.
Read more about [available default templates](../core-concepts/templates/available.md)
## Next Steps
* [Adding Sections](./adding-sections/overview.md)
---
---
url: /theme-editor/advanced-usage.md
---
# Advanced Usage
Once you're comfortable using the Visual Editor, there are a few advanced features and tips that can help you get even more control over your storefront.
## Layout vs. Page Content
Not all sections are the same:
* **Header and Footer sections** are part of the layout and appear on every page.
* **Main Content sections** are unique to the specific page you're editing (like the homepage or contact page).
Layout sections usually cannot be removed or reordered. They’re designed to stay consistent across the entire site.
## Working with Blocks
Some sections (like category grids or image banners) contain **blocks** — small pieces of content inside the section.
You can:
* Click a section to reveal its blocks
* Add new blocks (if allowed)
* Rearrange or delete them
* Customize each block’s settings (text, images, links, etc.)
## Preview Tools
At the top of the editor, you’ll find useful controls:
* **Device preview**: See how your page looks on desktop, tablet, and mobile
* **Language switcher**: If your store supports multiple languages, preview translations
* **Page selector**: Quickly switch between pages (homepage, contact, product, etc.)
## Best Practices
* **Edit in small steps** — Changes save automatically, so there's no need to rush.
* **Use meaningful section names** — It helps you and your team stay organized.
* **Keep it consistent** — Reuse similar section types across pages to create a cohesive design.
* **Preview before publishing** — Always test how things look on mobile and tablet before going live.
***
The Visual Editor is designed to be flexible and easy, while giving you the tools to make your storefront truly your own.
---
---
url: /core-concepts/architecture.md
---
# Anatomy of a Theme
Bagisto Visual brings a **modern, flexible theme system** to Bagisto — **heavily inspired by Shopify's proven architecture**.
At its core, a theme is a **structured collection of layouts, templates, sections, blocks, and settings**, allowing both developers and store owners to collaboratively build and manage storefronts with ease.
By adopting a system modeled after Shopify, Bagisto Visual empowers **merchants** and **theme developers** to take full control of the storefront experience — crafting **beautiful, fully customized online stores** that stand out from the competition.
This section explains **how a Bagisto Visual theme is organized**, **how the core parts fit together**, and **how developers and merchants can collaborate** to build dynamic, customizable storefronts.
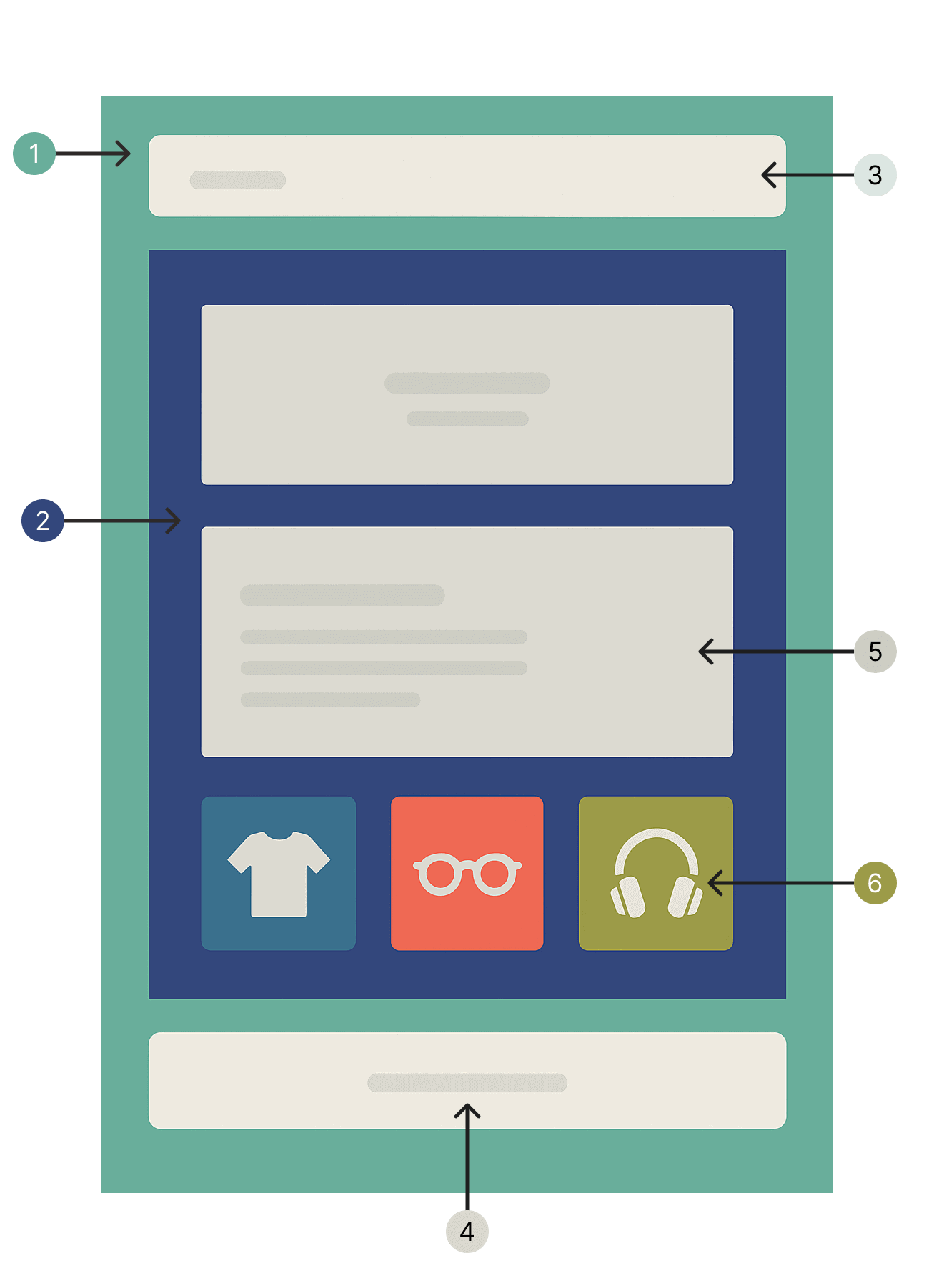
## Theme Structure
A theme is made up of the following main parts:
| Number | Part | Description |
| ------ | -------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| 1 | **Layout** | The base structure of the page. Defines global elements like header, footer, and meta. |
| 2 | **Template** | Define what to render and where. Each page of the storefront has its own associated template. Templates can be Blade (with dynamic logic) or JSON/YAML (as wrappers for sections). Only one template is rendered per page. |
| 3,4 | **Layout section** | Content blocks that are static in the layout. shared accross all pages |
| 5 | **Template section** | Modular, reusable content blocks used inside templates. |
| 6 | **Block** | smaller repeatable components inside sections. |
> **Note:**
> Templates define the structure of different page types in your storefront, such as the homepage, product pages, category pages, cart, and more. Each page is rendered based on its associated template, making it easy to create custom layouts for different parts of your store.
## Folder Structure
```plaintext
/theme/
├── resources/
│ ├── views/
│ ├── components/ # Custom Blade or Livewire components
│ ├── layouts/ # Layouts (default layout is mandatory)
│ ├── sections/ # Section Blade files
│ ├── templates/ # Page templates (JSON, YAML, or Blade)
├── src/
│ ├── Sections/ # PHP classes for custom sections
```
## Key Concepts
### Layouts
* Define the **global structure** of your pages.
* Must include a `default.blade.php` layout inside `/resources/views/layouts/`.
* Layouts typically contain the ``, header, footer, and dynamic content area.
### Templates
* Represent **individual pages** (e.g., homepage, product page).
* Can be written in **Blade**, **JSON**, or **YAML**.
* Templates **list sections** in the desired order.
* Only one template is rendered at a time based on the page being viewed.
### Sections
* **Reusable content blocks** like banners, product grids, and footers.
* Created using Blade templates located in `/resources/views/sections/`.
### Blocks (Component)
* **Sub-components** inside sections.
* Allow merchants to add, reorder, or remove content inside a section.
---
---
url: /core-concepts/templates/available.md
---
# Available Templates
Templates define the structure and behavior of pages in Bagisto Visual storefronts.
Each template determines:
* Which sections appear on the page
* Which data is available to sections
* How customers interact with the store
Bagisto Visual uses a flexible system where templates can be built with **Blade**, **JSON**, or **YAML** formats.
Each template may automatically expose **some variables** (like `$product`, `$category`, `$order`) to the sections it loads, making it easy to create dynamic, data-driven content.
## About This Page
This page documents:
* All available default templates provided by Bagisto Visual
* The variables each template shares with its sections
* Where templates are located in the theme directory
* Example usage of shared variables in Blade sections
## cart
The **Cart Template** displays the contents of a customer's shopping cart.
It is used to show the list of products that the customer has added to their cart, along with quantities, totals, and checkout options.
### Location
```plaintext
/theme/resources/views/templates/cart.yaml
```
### Shared variables
| Variable | Type | Description |
| -------- | ---------- | ----------------------------------- |
| $cart | Cart model | The current shopping cart instance. |
### Example
Example inside a section Blade file:
```blade
@if (isset($cart))
Cart subtotal: {{ core()->currency($cart->base_sub_total) }}
@endif
```
## category
The **Category Template** is used to display a list of products belonging to a specific category.
It typically includes features like filters, sorting options, product grids, and category banners.
### Location
```plaintext
/theme/resources/views/templates/category.json
```
### Shared variables
| Variable | Type | Description |
| --------- | -------------- | ------------------------------------- |
| $category | Category model | The currently viewed category object. |
### Example
Example inside a section Blade file:
```blade
@if (isset($category))
{{ $category->name }}
{{ $category->description }}
@endif
```
## checkout-success
The **Checkout Success Template** displays the order confirmation page after a successful checkout.
It shows the customer a summary of their completed order and any next steps or messages.
### Location
```plaintext
/theme/resources/views/templates/checkout-success.yaml
```
### Shared variables
| Variable | Type | Description |
| -------- | ----------- | ----------------------------------- |
| $order | Order model | The recently placed order instance. |
### Example
Example inside a section Blade file:
```blade
@if (isset($order))
Thank you for your order #{{ $order->id }}!
Total: {{ core()->currency($order->base_grand_total) }}
@endif
```
## checkout
The **Checkout Template** is used to display the checkout process, including customer information, shipping, and payment details.
It typically shows a summary of the cart and allows customers to complete their purchase.
### Location
```plaintext
/theme/resources/views/templates/checkout.yaml
```
### Shared variables
| Variable | Type | Description |
| -------- | ---------- | ----------------------------------- |
| $cart | Cart model | The current shopping cart instance. |
### Example
Example inside a section Blade file:
```blade
@if (isset($cart))
Cart subtotal: {{ core()->currency($cart->base_sub_total) }}
Number of items: {{ $cart->items->count() }}
@endif
```
## compare
The **Compare Template** is used to display a side-by-side comparison of selected products.
It allows customers to view product attributes and differences to help them make purchasing decisions.
### Location
```plaintext
/theme/resources/views/templates/compare.json
```
### Shared variables
| Variable | Type | Description |
| --------------------- | ----- | -------------------------------------------- |
| $comparableAttributes | array | Attributes available for product comparison. |
### Example
Example inside a section Blade file
```blade
@if (!empty($comparableAttributes))
Compare Products By:
@foreach ($comparableAttributes as $attribute)
- {{ $attribute['name'] }}
@endforeach
@endif
```
## error
The **Error Template** is used to display an error page when something goes wrong.
It shows an error message and error code based on what occurred (e.g., 404 not found, 500 server error).
### Location
```plaintext
/theme/resources/views/templates/error.blade.php
```
### Shared variables
| Variable | Type | Description |
| ---------- | ------- | -------------------- |
| $errorCode | integer | The HTTP error code. |
### Example
```blade
@if (isset($errorCode))
Error {{ $errorCode }}
Sorry, something went wrong.
@endif
```
## index
The **Index Template** is used to display the homepage of the storefront.
It typically features banners, featured collections, featured products, and custom landing page content.
### Location
```plaintext
/theme/resources/views/templates/index.yaml
```
### Shared variables
There are **no specific shared variables** automatically passed to sections in the index template.
Sections are responsible for fetching and displaying the homepage content themselves.
### Example
Since no variable is automatically exposed, a typical section might look like:
```blade
```
## page
The **Page Template** is used to render CMS pages created from the admin panel.
It displays static content like About Us, Contact, Terms, or any custom page.
### Location
```plaintext
/theme/resources/views/templates/page.yaml
```
### Shared variables
| Variable | Type | Description |
| -------- | ------------ | -------------------------- |
| $page | `Page` model | The CMS page being viewed. |
### Example
Example inside a section Blade file:
```blade
@if (isset($page))
{{ $page->title }}
{!! $page->content !!}
@endif
```
## product
The **Product Template** is used to display the details of a single product.
It includes the product title, description, images, price, reviews, and add-to-cart functionality.
### Location
```plaintext
/theme/resources/views/templates/product.json
```
### Shared variables
| Variable | Type | Description |
| -------- | --------------- | ----------------------------------- |
| $product | `Product` model | The product currently being viewed. |
### Example
Example inside a section Blade file:
```blade
@if (isset($product))
{{ $product->name }}
{{ $product->short_description }}
Price: {{ core()->currency($product->price) }}
@endif
```
## search
The **Search Template** is used to display search results based on a customer’s query.
It renders product listings that match keywords, tags, categories, or other filters.
### Location
```plaintext
/theme/resources/views/templates/search.json
```
### Shared variables
There are **no specific shared variables** exposed automatically by the search template.
Sections should retrieve search results using request query parameters or internal APIs.
### Example
A simple section might handle search results like this:
```blade
@php
$query = request()->get('term');
@endphp
Results for "{{ $query }}"
@livewire('search-results', ['term' => $query])
```
## auth/forgot-password
The **Forgot Password Template** displays a form that allows users to request a password reset link.
It is typically accessed from the login page if a user forgets their credentials.
### Location
```plaintext
/theme/resources/views/templates/auth/forgot-password.blade.php
```
### Shared variables
There are **no shared variables** exposed by this template.
### Example
```blade
```
## auth/login
The **Login Template** displays the customer login form.
It allows users to enter their email and password to access their account.
### Location
```plaintext
/theme/resources/views/templates/auth/login.blade.php
```
### Shared variables
There are **no shared variables** exposed by this template.
### Example
Example section for the login form:
```blade
```
## auth/register
The **Register Template** displays the customer registration form.
It allows new users to create an account by providing personal and login information.
### Location
```plaintext
/theme/resources/views/templates/auth/register.blade.php
```
### Shared variables
There are **no shared variables** exposed by this template.
### Example
Example registration form inside a section:
```blade
```
## auth/reset-password
The **Reset Password Template** displays a form to allow users to reset their password after receiving a reset link.
This page is accessed via the password reset email sent from the Forgot Password flow.
### Location
```plaintext
/theme/resources/views/templates/auth/reset-password.blade.php
```
### Shared variables
There are **no shared variables** exposed by this template.
### Example
Example form inside a section:
```blade
```
## account/add-address
The **Add Address Template** displays a form that allows customers to add a new address to their account.
It typically includes fields for name, address, city, country, and contact information.
### Location
```plaintext
/theme/resources/views/templates/account/add-address.blade.php
```
### Shared variables
There are **no shared variables** exposed by this template.
### Example
Example form inside a section:
```blade
```
## account/addresses
The **Addresses Template** displays a list of all addresses saved by a customer in their account.
It allows customers to view, edit, or delete their saved addresses.
### Location
```plaintext
/theme/resources/views/templates/account/addresses.blade.php
```
### Shared variables
There are **no shared variables** exposed by this template.
### Example
Example section to list addresses:
```blade
My Addresses
@foreach (auth('customer')->user()->addresses as $address)
{{ $address->address1[0] }}, {{ $address->city }}
{{ $address->state }}, {{ $address->country }}
{{ $address->phone }}
Edit
@endforeach
```
## account/downloadables
The **Downloadables Template** displays a list of downloadable products that a customer has purchased.
It allows customers to download digital files like e-books, software, or media after purchase.
### Location
```plaintext
/theme/resources/views/templates/account/downloadables.yaml
```
### Shared variables
There are **no shared variables** exposed by this template.
### Example
Example section for listing downloadable items
```blade
My Downloadable Products
@foreach (auth('customer')->user()->downloadable_products as $download)
@endforeach
```
## account/edit-address
The **Edit Address Template** displays a form that allows customers to update an existing address saved in their account.
### Location
```plaintext
/theme/resources/views/templates/account/edit-address.yaml
```
### Shared variables
| Variable | Type | Description |
| -------- | --------------- | ---------------------------------- |
| $address | `Address` model | The address instance being edited. |
### Example
Example section for editing an address:
```blade
```
## account/edit-profile
The **Edit Profile Template** displays a form that allows customers to update their personal account information, such as name, gender, date of birth, and email.
### Location
```plaintext
/theme/resources/views/templates/account/edit-profile.yaml
```
### Shared variables
There are **no special shared variables** passed directly into this template.
> Sections are expected to use `auth('customer')->user()` to retrieve and update the authenticated customer's data.
### Example
Example section for editing profile:
```blade
```
## account/order-details
The **Order Details Template** displays the complete details of a specific customer order.
It shows the order items, shipping address, billing address, totals, and current status.
### Location
```plaintext
/theme/resources/views/templates/account/order-details.yaml
```
### Shared variables
| Variable | Type | Description |
| -------- | ------------- | ---------------------------- |
| $order | `Order` model | The customer's order object. |
### Example
Example section to display order information:
```blade
Order #{{ $order->id }}
Order Date: {{ $order->created_at->format('M d, Y') }}
Total: {{ core()->currency($order->base_grand_total) }}
Items:
@foreach ($order->items as $item)
- {{ $item->name }} × {{ $item->qty_ordered }}
@endforeach
```
## accounts/orders
The **Orders Template** displays a list of all past orders placed by the customer.
Customers can view order summaries and access order details pages from here.
It typically uses a datagrid component to render the list dynamically, including pagination, filtering, and view links.
### Location
```plaintext
/theme/resources/views/templates/account/orders.yaml
```
### Shared variables
There are **no shared variables** exposed by this template.
> Customer orders are typically listed using bagisto datagrid component or retrieved inside sections manually.
### Example
Example section for embedding a datagrid component
```blade
```
Or a minimal manual fetch:
```blade
My Orders
@foreach (auth('customer')->user()->orders as $order)
Order #{{ $order->id }} placed on {{ $order->created_at->format('M d, Y') }}
Total: {{ core()->currency($order->base_grand_total) }}
View Details
@endforeach
```
## account/profile
The **Profile Template** displays the customer's personal information such as name, email, and contact details.
It also typically provides options to update profile information or change the password.
### Location
```plaintext
/theme/resources/views/templates/account/profile.yaml
```
### Shared variables
There are **no shared variables** exposed by this template.
> Sections are expected to use `auth('customer')->user()` to access the currently authenticated customer's profile information.
### Example
Example section to display the customer profile:
```blade
My Profile
Name: {{ auth('customer')->user()->first_name }} {{ auth('customer')->user()->last_name }}
Email: {{ auth('customer')->user()->email }}
Edit Profile
```
## account/reviews
The **Reviews Template** displays all product reviews submitted by the customer.
It shows the products reviewed, ratings given, and review comments.
### Location
```plaintext
/theme/resources/views/templates/account/reviews.yaml
```
### Shared variables
| Variable | Type | Description |
| -------- | ---------------------------- | ---------------------------------------------- |
| $reviews | Collection of `Review` model | The list of reviews submitted by the customer. |
### Example
Example section to list customer reviews:
```blade
My Reviews
@foreach ($reviews as $review)
{{ $review->product->name }}
Rating: {{ $review->rating }} / 5
{{ $review->comment }}
@endforeach
```
## account/wishlist
The **Wishlist Template** displays the products that a customer has added to their wishlist.
Customers can view, manage, and move wishlist items to the cart.
### Location
```plaintext
/theme/resources/views/templates/account/wishlist.yaml
```
### Shared variables
There are **no shared variables** exposed by this template.
### Example
Example section to display wishlist items:
```blade
My Wishlist
@foreach (auth('customer')->user()->wishlist_items as $item)
{{ $item->product->name }}
Remove
@endforeach
```
---
---
url: /building-theme/best-practices/overview.md
---
# Best Practices for building theme
Bagisto Visual gives developers a powerful and flexible system for building customizable storefronts. But with flexibility comes the need for consistency and thoughtful design.
This section outlines best practices for creating maintainable, accessible, and performant visual themes and sections.
Whether you're building a full theme or just a single reusable section, these guidelines will help ensure your work:
* Integrates smoothly with the visual editor
* Adheres to accessible and semantic markup
* Works reliably across templates and devices
* Supports theme-level customization and branding
## Topics Covered
* [Styling and Color System](./styling.md)
Use the shared design token system to ensure theme compatibility and customization.
* [Accessibility](./accessibility.md)
Build for users of all abilities with semantic HTML and keyboard support.
* [Performance](./performance.md)
Deliver lightweight, responsive sections and themes.
***
Following these practices helps you build better themes — and helps merchants build better stores.
---
---
url: /building-theme/create-theme.md
---
# Creating a New Theme
Bagisto Visual makes it easy to create a new theme using a single command.
## Step 1: Generate a Theme
To scaffold a brand new theme, run the following Artisan command:
```bash
php artisan visual:make-theme "Awesome Theme"
```
By default, the theme will be placed under the `Themes` vendor namespace.
***
### Optional: Customize the Vendor Name
You can also customize the vendor (namespace) if needed:
```bash
php artisan visual:make-theme "Awesome Theme" --vendor="MyVendor"
```
This will generate the theme inside:
```text
src/Packages/MyVendor/AwesomeTheme/
```
If the `--vendor` option is not provided, it defaults to:
```text
src/Packages/Themes/AwesomeTheme/
```
✅ This is useful if you're developing themes under different brand or client namespaces.
## Step 2: Theme Directory Structure
Here’s what a freshly generated theme looks like:
```text
AwesomeTheme/
├── config/
│ ├── settings.php # Global theme settings (colors, fonts, social links, etc.)
│ └── theme.php # Main theme metadata (name, author, version)
├── resources/
│ ├── assets/
│ │ └── images/
│ │ └── theme-preview.png # Theme preview image
│ └── views/
│ ├── components/ # Blade components used inside sections
│ ├── layouts/ # Main layouts (default.blade.php, account.blade.php)
│ ├── sections/ # Blade views for each section
│ └── templates/ # Page templates (Blade, JSON, or YAML)
├── src/
│ ├── Sections/ # PHP classes defining sections and blocks
│ │ ├── ExampleSection.php
│ │ └── ...
│ └── ServiceProvider.php # Registers the theme into Bagisto Visual
├── package.json # Frontend dependencies and build scripts
├── composer.json # PHP package metadata for autoloading
├── tailwind.config.js # TailwindCSS configuration (optional)
├── vite.config.ts # ViteJS configuration for assets
└── README.md # Theme documentation (optional)
```
✅
The `theme-preview.png` file will be used to visually represent your theme in the Theme Editor.
### Understanding Important Files
#### `config/theme.php`
Defines core metadata and configuration for your theme:
```php
"awesome-theme",
"name" => "Awesome Theme",
"version" => "1.0.0",
"author" => "Your Company Name",
"assets_path" => "public/themes/shop/awesome-theme",
"views_path" => "resources/themes/awesome-theme/views",
"preview_image" => "public/themes/shop/awesome-theme/preview.png",
"documentation_url" => "https://yourdomain.com/docs/themes/awesome-theme",
"vite" => [
"hot_file" => "awesome-theme-vite.hot",
"build_directory" => "themes/awesome-theme/dist",
"package_assets_directory" => "resources/assets"
]
];
```
#### `config/settings.php`
Defines editable theme-level settings such as:
* Colors
* Fonts
* Header and footer links
* Social icons
These settings are exposed in the Theme Editor and configurable by merchants.
#### `resources/views/layouts/`
Contains layout Blade files like `default.blade.php`.
All pages render inside one of these layouts.
#### `resources/views/templates/`
Templates define full pages and reference a layout and a list of sections.
They can be Blade, JSON, or YAML.
#### `resources/views/sections/`
Contains section Blade files.
Each section is a reusable UI block that can be added to templates in the visual editor.
### Starting From a Starter Theme
By default, the scaffold generates a **blank theme** with no layout, sections, or styles.
If you prefer to start with a fully built base theme, you can clone the official Bagisto Visual starter theme:
```bash
git clone https://github.com/bagistoplus/visual-debut packages/Themes/YourThemeName
```
Then, update the `composer.json` and `config/theme.php` files to reflect your own theme name and vendor.
✅
This gives you a complete, working theme based on Visual Debut, which you can customize freely — layout, colors, sections, templates, or styles.
## Step 3: Installing and Using the Theme
Once the theme is generated, it is automatically configured inside your Bagisto project using Composer's local repository system.
You can immediately install the theme by running:
```bash
composer require themes/awesome-theme
```
✅
No need to publish the theme package to Packagist or any remote server.
You only need to publish the package if you intend to distribute or share it outside your current project.
## Step 4: Preview the Theme in Admin
After installation, activate your theme in the admin panel:
1. Log into the Bagisto admin panel.
2. Go to **Bagisto Visual → Themes**.
3. You should see the newly installed theme listed.
4. Click on the **Preview** button to see a preview of the theme.
5. Optional: click of the **Customize** button to open the theme in visual editor.
✅
You can start working on your theme now.
## Next Steps
Once your theme is generated, you can continue with:
* [Adding Layouts](./adding-layouts.md)
* [Creating Templates](./adding-templates.md)
* [Creating Sections](./adding-sections/overview.md)
* [Configuring Theme Settings](../core-concepts/settings/theme-settings.md)
---
---
url: /building-theme/adding-sections/creating-section.md
---
# Creating a Section
A section is a configurable UI component used to compose templates in Bagisto Visual.
Basically a section is just a Blade or Livewire component that defines structure, behavior, settings, and rendering logic.
## Generate a Section
To generate a new section, use the `visual:make-section` Artisan command:
```bash
php artisan visual:make-section AnnouncementBar --theme=awesome-theme
```
This will create a basic Blade section class named AnnouncementBar inside the awesome-theme package:
```text
packages/Themes/AwesomeTheme/src/Sections/AnnouncementBar.php
```
Generated class content:
```php
Announcement Bar
```
::: info
Ensure the theme is already installed:
```bash
composer require themes/awesome-theme
```
:::
### `--livewire`
Use this flag to generate a Livewire-based section:
```bash
php artisan visual:make-section AnnouncementBar --livewire --theme=awesome-theme
```
This creates a class that extends LivewireSection and includes a Livewire component stub.
### No `--theme`
If omitted, the section is placed in:
```text
app/Visual/Sections/AnnouncementBar.php
```
## Registering Sections
Bagisto Visual automatically discovers sections from:
* `app/Visual/Sections`
* `packages/